22
|
Is it possible to let user choose the inserting mode, when he presses Insert key ( method 1 )
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L"00:00");
spMaskEdit1->PutText(L"12:3");
spMaskEdit1->PutAllowToggleInsertMode(VARIANT_TRUE);
spMaskEdit1->PutInsertMode(EXMASKEDITLib::exEditOvertypeMode);
|
21
|
Does your control support overtype mode ( method 2 )

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L"00:00;;;overtype");
spMaskEdit1->PutText(L"12:3");
|
20
|
Does your control support overtype mode ( method 1 )

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutInsertMode(EXMASKEDITLib::exEditOvertypeMode);
spMaskEdit1->PutMask(L"00:00");
spMaskEdit1->PutText(L"12:3");
|
19
|
How can I change the colors to show a read only field
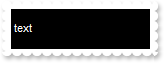
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutForeColorReadOnly(RGB(255,255,255));
spMaskEdit1->PutBackColorReadOnly(RGB(0,0,0));
spMaskEdit1->PutReadOnly(VARIANT_TRUE);
spMaskEdit1->PutText(L"text");
|
18
|
How can I lock or make read only the field (method 2)

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutReadOnly(VARIANT_TRUE);
spMaskEdit1->PutText(L"text");
|
17
|
How can I lock or make read only the field (method 1)

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L"*;;;readonly");
spMaskEdit1->PutText(L"text");
|
16
|
Is it possible to mask a password field (method 2)
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutRight(VARIANT_TRUE);
spMaskEdit1->PutText(L"text");
|
15
|
Is it possible to right align field (method 1)
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L"*;;;right");
spMaskEdit1->PutText(L"text");
|
14
|
Is it possible to mask a password field (method 2)

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutPassword(VARIANT_TRUE);
spMaskEdit1->PutText(L"password");
|
13
|
Is it possible to mask a password field (method 1)

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L"*;;;password");
spMaskEdit1->PutText(L"password");
|
12
|
How can I mask an integer within a range

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L"{1950,2050}");
spMaskEdit1->PutText(L"1979");
|
11
|
How can I mask an integer value with no grouping support

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L";;;float,grouping=,decimal=,digits=0,select=1");
spMaskEdit1->PutText(L"12345.67");
|
10
|
How can I mask an integer value (method 2)

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L"-#####;;;float,select=1");
spMaskEdit1->PutText(L"-12345.67");
|
9
|
How can I mask an integer value (method 1)

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L";;;float,decimal=,digits=0,select=1");
spMaskEdit1->PutText(L"12345.67");
|
8
|
How can I specify the number of digits when masking a float (method 2)

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L"###.#;;;float,select=1");
spMaskEdit1->PutText(L"12345.67");
|
7
|
How can I specify the number of digits when masking a float (method 1)

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L";;;float,select=1,digits=1");
spMaskEdit1->PutText(L"12345.67");
|
6
|
How do I mask a positive, floating point numbers support, including grouping of digits

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L";;;float,select=1,negative=0");
spMaskEdit1->PutText(L"-12345.67");
|
5
|
How do I mask a floating point numbers support, with a different decimal character

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L";;;float,grouping= ,decimal=\\,,select=1");
spMaskEdit1->PutText(L"12345,67");
|
4
|
How do I mask a floating point numbers support, excluding grouping of digits

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L";;;float,grouping=,select=1");
spMaskEdit1->PutText(L"12345.67");
|
3
|
How do I mask a floating point numbers support, including grouping of digits

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutMask(L";;;float,select=1");
spMaskEdit1->PutText(L"12345.67");
|
2
|
How can I change the control's foreground color

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutForeColor(RGB(255,0,0));
spMaskEdit1->PutText(L"aka");
|
1
|
How can I change the control's background color
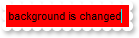
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXMASKEDITLib' for the library: 'ExMaskEdit 7.1 Control Library'
#import <MaskEdit.dll>
using namespace EXMASKEDITLib;
*/
EXMASKEDITLib::IMaskEditPtr spMaskEdit1 = GetDlgItem(IDC_MASKEDIT1)->GetControlUnknown();
spMaskEdit1->PutBackColor(RGB(255,0,0));
|